How To Find Lower Endpoint And Upper Endpoint
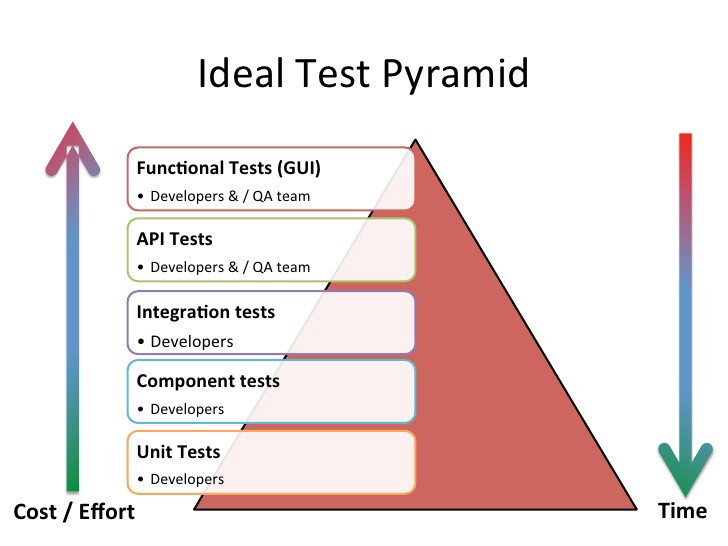
past Emre Savcı
How to examination services, endpoints, and repositories in Spring Boot
In this post I will show you how to write unit tests in spring kick applications.
Why is it necessary to write unit test requires some other commodity to explicate. Just for a cursory explanation, I will tell you several things.
I commonly defend the argument that code without unit tests is dead code. Considering, when a developer adds a new characteristic to some code which is non covered by a unit of measurement exam, information technology is decumbent to override existing business rules (which kills the code written before). Maybe it's not exactly decumbent to it, only you lot tin imagine what errors tin occur when a complex projection needs to exist changed. Unit testing is the only mode to protect your lawmaking against breaking changes.
Why unit examination endpoints?
Every time nosotros write an endpoint we need to be certain several things work correctly. The endpoint should return the information in the correct structure and handle the request correctly. We can test it manually, which is non preferable. So we write unit tests to ensure that our endpoints piece of work correctly. At that place is also another way for testing endpoints known as automation tests, merely that is non the subject of this mail service.
Why unit test services?
It should exist clear already, but but in case: nosotros demand to be sure our business concern logic works correctly.
Why unit test repositories?
There are several cases to test repositories. Of grade we don't examination the framework itself. But we practise write unit of measurement tests to be certain that our specifications or relations have been implemented correctly.
So how practise we test controllers?
Now information technology's time to bear witness you how to test our controllers in jump boot. Let'southward imagine we write an awarding which allows united states to save users in a database. We define a user entity, a user service, and a controller.
Annotation: The examples shown in this post are non for real product employ architecture
@Data@Entitypublic class User { @Id @GeneratedValue(generator = "uuid2") @GenericGenerator(name = "uuid2", strategy = "org.hibernate.id.UUIDGenerator") @Column(name = "id", columnDefinition = "BINARY(sixteen)") individual UUID id; private Cord name; individual String email; private int age;}
@Datapublic class CreateUserRequest { private Cord name; private String electronic mail; private int age;}
@RestController@RequestMapping("/users")public class UserController { UserService userService; @Autowired public UserController(UserService userService) { this.userService = userService; } @PostMapping public ResponseEntity<User> createUser(@RequestBody CreateUserRequest request) { User created = userService.save(request); render ResponseEntity.ok(created); }}
Our controller has a dependency on UserService merely we aren't interested in what service does correct now.
So now allow's write a unit test for our controller to be sure it works correctly.
We mocked our service because we don't need its implementation details. We just examination our controller here. We use MockMvc
here to examination our controller and object mapper for serialization purposes.
We setup our userService.Save()
method to return the desired user object. We passed a asking to our controller and after that we checked the returned data with the following line: andExpect(jsonPath("$.name").value(asking.getName()))
.
We have also other methods to use. Here is the list of methods:
When nosotros run the test we see that it passes.
How practice nosotros test services?
Now we go to test our UserService. It is quite simple to test.
We mock the repository and inject our mocks into UserService. At present when we run the test we'll come across that it passes.
Now let's add a business dominion to UserService: allow's say the user must have an email address.
We change our save method in UserService equally below:
public User salvage(CreateUserRequest request) { requireNonNull(request.getEmail()); User user = new User(); user.setName(request.getName()); user.setEmail(request.getEmail()); user.setAge(request.getAge()); userRepository.relieve(user); render user;}
When we run the test again, we'll run across a failed test.
Before we set it, allow'south write a exam that satisfies this business organization.
We wrote a new test that specified that if we send a naught email, information technology'll throw NullPointerException.
Let's fix the failed test by adding an electronic mail to our request:
createUserRequest.setEmail("testemail");
Run both tests:
How practise we test repositories?
Now nosotros've come to testing repositories. We utilize an in retention h2 database with TestEntityManager.
Our repository is divers as below:
@Repositorypublic interface UserRepository extends JpaRepository<User, Integer>, JpaSpecificationExecutor<User> { Optional<User> findById(UUID id);}
Start configure h2db. Create the file name application.yaml in examination -> resources path:
spring: awarding: proper noun: Spring Boot Remainder API datasource: type: com.zaxxer.hikari.HikariDataSource url: "jdbc:h2:mem:test-api;INIT=CREATE SCHEMA IF NOT EXISTS dbo\\;CREATE SCHEMA IF NOT EXISTS definitions;DATABASE_TO_UPPER=false;DB_CLOSE_DELAY=-one;DB_CLOSE_ON_EXIT=simulated;MODE=MSSQLServer" name: password: username: initialization-mode: never hikari: schema: dbo jpa: database: H2 database-platform: org.hibernate.dialect.H2Dialect show-sql: true hide: ddl-auto: create-driblet test: database: supercede: none
And let's commencement write a basic test for our repository: salve a user and call up it:
@RunWith(SpringRunner.class)@DataJpaTestpublic form UserRepositoryTest { @Autowired TestEntityManager entityManager; @Autowired UserRepository sut; @Test public void it_should_save_user() { User user = new User(); user.setName("test user"); user = entityManager.persistAndFlush(user); assertThat(sut.findById(user.getId()).get()).isEqualTo(user); }}
When we run it we'll run across bunch of panel output, and also our test passes:
Now let'due south add another method to our repository for searching for a user via email:
Optional<User> findByEmail(String e-mail);
And write some other test:
@Testpublic void it_should_find_user_byEmail() { User user = new User(); user.setEmail("testmail@test.com"); user = entityManager.persistAndFlush(user); assertThat(sut.findByEmail(user.getEmail()).get()).isEqualTo(user);}
When we accept a wait at the console later on running the exam, we'll run into the SQL generated by hibernate:
SELECT user0_.id AS id1_1_,user0_.age AS age2_1_,user0_.e-mail Every bit email3_1_,user0_.name AS name4_1_FROM user user0_WHERE user0_.email=?
So far and then good. Nosotros have covered the basics of unit of measurement testing with spring boot.
Now y'all don't accept any excuses to not write unit tests! I hope it is articulate to you to how to write unit tests for dissimilar kinds of purposes.
Learn to lawmaking for costless. freeCodeCamp's open up source curriculum has helped more than than 40,000 people go jobs as developers. Become started
Source: https://www.freecodecamp.org/news/unit-testing-services-endpoints-and-repositories-in-spring-boot-4b7d9dc2b772/
Posted by: ungerloped1957.blogspot.com
0 Response to "How To Find Lower Endpoint And Upper Endpoint"
Post a Comment